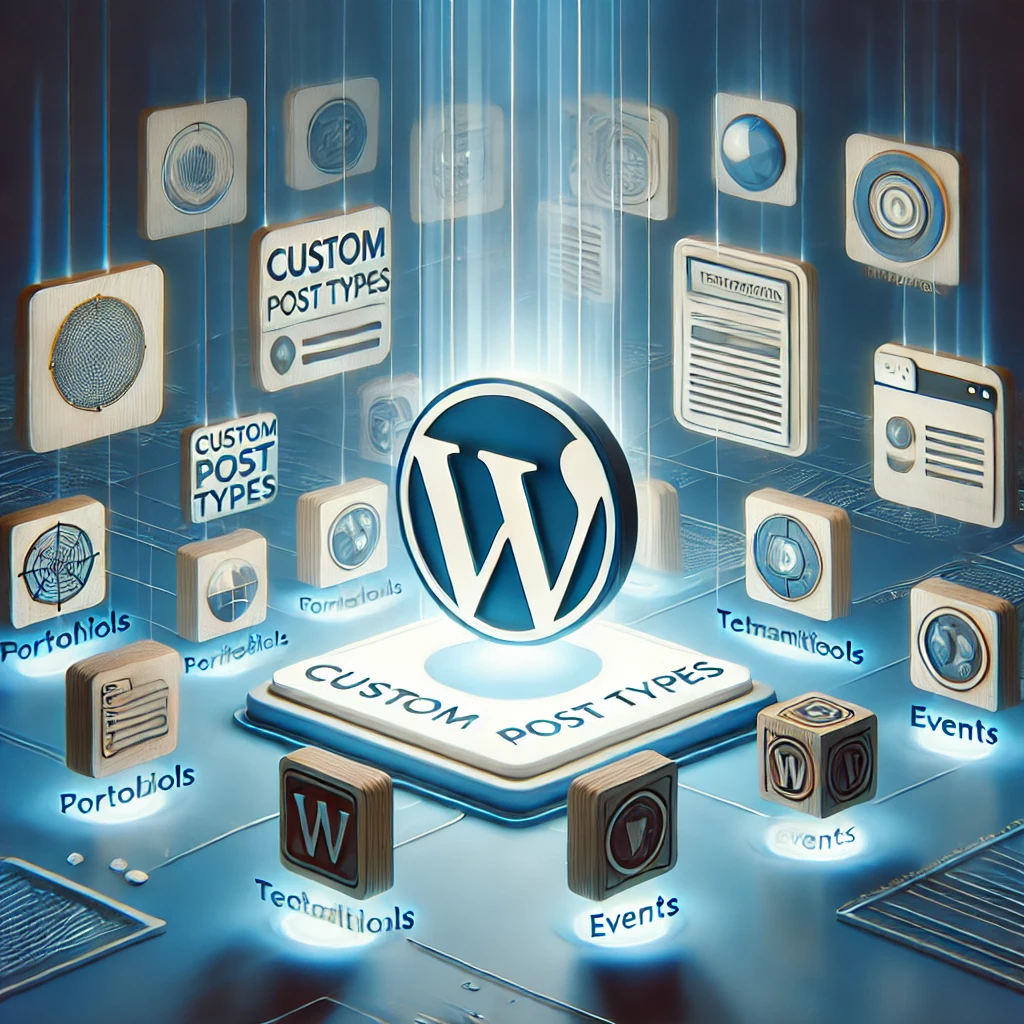
By default WordPress has some post type already but if we want to add new post type like if we want to add products in our cms so we need to create a custom post type names as “product” or as you wish.
There are some default post type are :
- Post
- Page
- Attachment
- Revision
- Nav Menu
When we create new post type then a separate menu section will create in wp-admin like Posts, Media so for this we need to add some codes for support custom post type and will appeare in wp-admin.
We can add custom post type(CPT) in two ways
- Using Plugin
- Using WordPress codes
1 .Using Plugin — We need to install Custom Post Type UI Plugin from wordpress.org and its free plugin.
When we install and activate this plugin then a menu will add in wp-admin “CPT UI”. Now click on CPT UI >> Add new then fill some details for CPT.
1. Slug — For url and WordPress quires for CPT
2. Plural label
3. Singular label
4. Other optional value
5. Click “Add Post Type”
2. Using WordPress Codes — We need to add codes in functions.php file. We need to use init hook.
Lets now we will create our CPT named as “product”
// Our custom post type function function pg_custom_post_type() { register_post_type( 'product', // CPT Options array( 'labels' => array( 'name' => __( 'Products' ), 'singular_name' => __( 'Product' ) ), 'public' => true, 'has_archive' => true, 'rewrite' => array('slug' => 'product'), 'show_in_rest' => true, ) ); } // Hooking up our function to theme setup add_action( 'init', 'pg_custom_post_type' );
Lets see more option for register_post_type() and will add in our code
/* * Creating a function to create our CPT */ function pg_custom_post_type() { // Set UI labels for Custom Post Type $labels = array( 'name' => _x( 'Products', 'Post Type General Name', 'phpguruji' ), 'singular_name' => _x( 'Product', 'Post Type Singular Name', 'phpguruji' ), 'menu_name' => __( 'Products', 'phpguruji' ), 'parent_item_colon' => __( 'Parent Product', 'phpguruji' ), 'all_items' => __( 'All Products', 'phpguruji' ), 'view_item' => __( 'View Product', 'phpguruji' ), 'add_new_item' => __( 'Add New Product', 'phpguruji' ), 'add_new' => __( 'Add New', 'phpguruji' ), 'edit_item' => __( 'Edit Product', 'phpguruji' ), 'update_item' => __( 'Update Product', 'phpguruji' ), 'search_items' => __( 'Search Product', 'phpguruji' ), 'not_found' => __( 'Not Found', 'phpguruji' ), 'not_found_in_trash' => __( 'Not found in Trash', 'phpguruji' ), ); // Set other options for Custom Post Type $args = array( 'label' => __( 'Product', 'phpguruji' ), 'description' => __( 'Product management', 'phpguruji' ), 'labels' => $labels, // Features this CPT supports in Post Editor 'supports' => array( 'title', 'editor', 'excerpt', 'author', 'thumbnail', 'comments', 'revisions', 'custom-fields', ),</pre> <pre> // You can associate this CPT with a taxonomy or custom taxonomy. 'taxonomies' => array( 'product_cat' ), /* A hierarchical CPT is like Pages and can have * Parent and child items. A non-hierarchical CPT * is like Posts. */ 'hierarchical' => false, 'public' => true, 'show_ui' => true, 'show_in_menu' => true, 'show_in_nav_menus' => true, 'show_in_admin_bar' => true, 'menu_position' => 5, 'can_export' => true, 'has_archive' => true, 'exclude_from_search' => false, 'publicly_queryable' => true, 'capability_type' => 'post', 'show_in_rest' => true, ); // Registering your Custom Post Type register_post_type( 'product', $args ); } add_action( 'init', 'pg_custom_post_type', 0 );
In given array, we can see many options in $args array like supports key has array of value which means our CPT will have all these extra features in our CPT like editor, thumbnail, comments, revisions etc.
As you can see, we use phpguruji string it is use for text-domain for localization.